π‘ 1. νλ‘νΌν° (Property)
- νλ‘νΌν°λ μμ±μ΄λΌλ λ»μΌλ‘, μλ°μ€ν¬λ¦½νΈμμλ κ°μ²΄ λ΄λΆμ μμ±μ μλ―Έν©λλ€.
let person = {
name : 'bigone',
age : 30
}
- μ μμλ₯Ό 보면 personμ΄λΌλ κ°μ²΄ μμ name, ageμ΄λΌλ keyκ° μλλ°, μ΄λ₯Ό νλ‘νΌν°λΌκ³ ν©λλ€.
π‘ 2. ν¨μ νλ‘νΌν°
- ν¨μλ κ°μ²΄μ΄λ―λ‘ νλ‘νΌν°λ₯Ό κ°μ§κ² λ©λλ€.
function sum (a, b){
return a + b;
}
console.dir(sum)
- sum ν¨μ μμ± μ½λλ₯Ό 보면 argument, caller λ±κ³Ό κ°μ νλ‘νΌν°λ€μ μμ±ν μ μ΄ μλλ°, μλμΌλ‘ μμ±μ΄ λμ΄μμ΅λλ€. μ΄λ Function μμ±μμ μν΄μ ν¨μκ° μμ±λ λ μλμΌλ‘ ν λΉμ΄ λ©λλ€.
- ν¨μμλ κΈ°λ³Έμ μΌλ‘ arguments, caller, length, name, prototype λ±μ νλ‘νΌν°κ° μμ΅λλ€.
π£ 2.1 arguments νλ‘νΌν°
- ν¨μ νΈμΆ μ μ λ¬λλ μΈμλ€μ μ 보λ₯Ό λ΄κ³ μλ λ°°μ΄μ λλ€. ν¨μ μμμλ§ μ¬μ© κ°λ₯ν μ§μλ³μμ λλ€.
function minus(a, b){
console.log(arguments);
return a-b;
}
minus(); // Arguments [callee: ƒ, Symbol(Symbol.iterator): ƒ]
minus(1); // Arguments [1, callee: ƒ, Symbol(Symbol.iterator): ƒ]
minus(2,1); // Arguments(2) [2, 1, callee: ƒ, Symbol(Symbol.iterator): ƒ]
minus(3,2,1); // Arguments(3) [3, 2, 1, callee: ƒ, Symbol(Symbol.iterator): ƒ]
- arguments νλ‘νΌν°λ μΌλΆ λΈλΌμ°μ μμ μ§μμ νκ³ μμΌλ, deprscated(μ€μλκ° λ¨μ΄μ Έ λ μ΄μ μ¬μ©λμ§ μκ³ μμΌλ‘ μ¬λΌμ§κ² λ )λμ΄ μ€λ¬΄μμλ κ±°μ μ¬μ©λκ³ μμ§ μμ΅λλ€.
π£ 2.2 caller νλ‘νΌν°
- caller νλ‘νΌν°λ μμ μ νΈμΆν ν¨μλ₯Ό μλ―Έν©λλ€.
function sum (a, b){
console.log(sum.caller);
return a+b;
}
function calculator(x, y) {
let sum_ = sum(x, y);
return sum_;
}
calculator(1, 10); // f calculator(x,y) {...}
sum(1,2); // null -> λΈλΌμ°μ μμ μ€ννμΌλ―λ‘ callerκ° μλ€.
- calculatorλ₯Ό ν΅ν΄μ sumν¨μλ₯Ό νΈμΆ μ sumμ callerμλ calculatorκ° λ€μ΄κ° μμ΅λλ€.
- sum ν¨μλ₯Ό μ§μ νΈμΆ μ callerλ nullμ λλ€. νΈμΆν ν¨μκ° μκΈ° λλ¬Έμ λλ€.
π£ 2.3 length νλ‘νΌν°
- length νλ‘νΌν°λ ν¨μ μ μ μ μμ±λ λ§€κ°λ³μ κ°μλ₯Ό μλ―Έν©λλ€.
// λ§€κ°λ³μ 0κ°
function func1(){}
// λ§€κ°λ³μ 1κ°
function func2(a){}
// λ§€κ°λ³μ 2κ°
function func3(a,b){}
console.log(func1.length); // 0
console.log(func2.length); // 1
console.log(func3.length); // 2
π£ 2.4 name νλ‘νΌν°
- ν¨μλͺ μ μλ―Έν©λλ€. κΈ°λͺ ν¨μ(μ΄λ¦μ λͺ μν ν¨μ)μ κ²½μ° ν¨μλͺ μ κ°μΌλ‘ κ°κ³ μ΅λͺ ν¨μμΈ κ²½μ° λΉ λ¬Έμμ΄μ κ°μΌλ‘ κ°κ² λ©λλ€. λ¨, ES6μ΄νλΆν°λ μ΅λͺ ν¨μμ μ΄λ¦μ΄ nameμ κ°μΌλ‘ ν λΉλ©λλ€.
// κΈ°λͺ
ν¨μ
function sum(a,b) {
return a+b;
}
// μ΅λͺ
ν¨μ
let minus = function(a,b) {
return a-b;
}
console.log(sum.name); // sum
console.log(minus.name); // ES6 μ΄μ -> '' , ES6λΆν° -> minus
π£ 2.5 __proto__ μ κ·Όμ νλ‘νΌν°
- λͺ¨λ κ°μ²΄λ [[Prototype]]μ΄λΌλ λ΄λΆ μ¬λ‘―μ΄ μκ³ , νλ‘ν νμ κ°μ²΄λ₯Ό κ°λ¦¬ν΅λλ€. νλ‘ν νμ κ°μ²΄λ κ°μ²΄ κ°μ μμ(Inheritance)μ ꡬννκΈ° μν΄ μ¬μ©λ©λλ€. (μ¬κΈ°μ μμμ μ½κ² λ§ν΄, λΆλͺ¨λμ΄ μμμκ² μ¬μ°μ μμνλ―μ΄ λΆλͺ¨ κ°μ²΄κ° μμ κ°μ²΄μκ² λΆλͺ¨μ μμ±μ μ£Όλ κ²μ μλ―Έν©λλ€.)
- __proto__ νλ‘νΌν°λ [[Prototype]] λ΄λΆ μ¬λ‘―μ΄ κ°λ¦¬ν€λ νλ‘ν νμ κ°μ²΄μ μ κ·Όνλ μ κ·Ό νλ‘νΌν°μ λλ€. λ΄λΆ μ¬λ‘―μλ μ§μ μ κ·Όμ ν μ μμΌλ―λ‘ __proto__λ₯Ό μ¬μ©νλ κ²μ λλ€.
console.log(Object.prototype) // {constructor: ƒ, __defineGetter__: ƒ, __defineSetter__: ƒ, hasOwnProperty: ƒ, __lookupGetter__: ƒ, …}
let obj = {};
console.log(obj.__proto__); // {constructor: ƒ, __defineGetter__: ƒ, __defineSetter__: ƒ, hasOwnProperty: ƒ, __lookupGetter__: ƒ, …}
console.log(Object.prototype === obj.__proto__); // true
- __proto__ νλ‘νΌν°λ κ°μ²΄κ° μ§μ μμ ν κ²μ΄ μλκ³ Object.prototype κ°μ²΄μ νλ‘νΌν°λ₯Ό μμλ°μ κ²μ λλ€.
// Object.prototype κ°μ²΄μ μλ‘μ΄ νλ‘νΌν° μΆκ°
Object.prototype.hello = function(){
console.log('Hi');
}
let a = {};
console.log(a.__proto__.hello); //ƒ (){console.log('Hi');}
π£ 2.6 prototype νλ‘νΌν°
- prototype νλ‘νΌν°λ ν¨μ κ°μ²΄μλ§ μλ νλ‘νΌν°μ λλ€. μΌλ° κ°μ²΄μλ prototype νλ‘νΌν°κ° μμ΅λλ€.
let a = {};
let b = function(){};
console.log(a.prototype); // undefined
console.log(b.prototype); // {constructor: ƒ}
- prototype νλ‘νΌν°λ μμ±μ ν¨μμ μν΄μ ν¨μλ₯Ό μμ±ν λ μ¬μ©λ©λλ€.
function Puppy (name) {
this.name = name;
}
Puppy.prototype = {
κ·μ¬μ : true
}
let κΎΈκΈ° = new Puppy('κΎΈκΈ°');
console.log(κΎΈκΈ°); // Puppy {name: 'κΎΈκΈ°'}
console.log(κΎΈκΈ°.κ·μ¬μ); // true
π μ 리
- νλ‘νΌν°λ κ°μ²΄μ μμ±μ μλ―Ένλ€.
- ν¨μ νλ‘νΌν°μλ κΈ°λ³Έμ μΌλ‘ arguments, caller, length, name, prototype νλ‘νΌν°κ° μλ€.
- arguments νλ‘νΌν°λ ν¨μκ° νΈμΆλ λ μ λ¬λ μΈμλ₯Ό λ΄μ λ°°μ΄
- callerλ ν¨μλ₯Ό νΈμΆν ν¨μ
- lengthλ ν¨μμ λ§€κ°λ³μμ κ°μλ₯Ό μλ―Έ
- prototypeμ ν¨μ κ°μ²΄μλ§ μκ³ , μμ±μ ν¨μλ‘ κ°μ²΄λ₯Ό μμ±ν λ μ¬μ©
κΈ΄ κΈ μ½μ΄μ£Όμ
μ κ°μ¬ν©λλ€ :)
νλ¦° λ΄μ©μ΄ μκ±°λ, λ§λΆμΌ λ΄μ©μ΄ μλ€λ©΄ μΈμ λ μ§ λκΈ λ¬μμ£ΌμΈμ!
μ κΈμ΄ μ‘°κΈμ΄λλ§ μ½μΌμ λΆλ€μκ² λμμ΄ λλλ‘ λ
Έλ ₯νκ² μ΅λλ€
λ€μ νΈμ 봬μ~
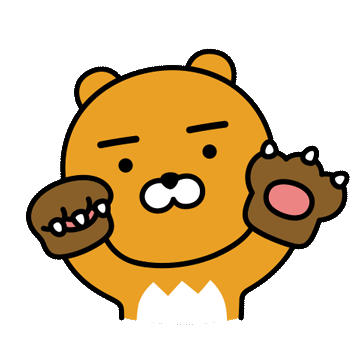
'Front-End > JavaScript' μΉ΄ν κ³ λ¦¬μ λ€λ₯Έ κΈ
[μλ°μ€λ¦½νΈ(JavaScript )] 13. Strict mode (0) | 2022.03.15 |
---|---|
[μλ°μ€λ¦½νΈ(JavaScript )] 12. ν¨μμ λ€μν νν (μ¦μ μ€ν ν¨μ, λ΄λΆ ν¨μ, callback λ±) (0) | 2022.02.21 |
[μλ°μ€λ¦½νΈ(JavaScript )] 10. ν¨μ (Function) (0) | 2022.02.04 |
[μλ°μ€λ¦½νΈ(JavaScript )] 09. κ°μ²΄ (Object) (0) | 2022.02.03 |
[JavaScript] 08. νμ λ³ν (0) | 2022.01.27 |